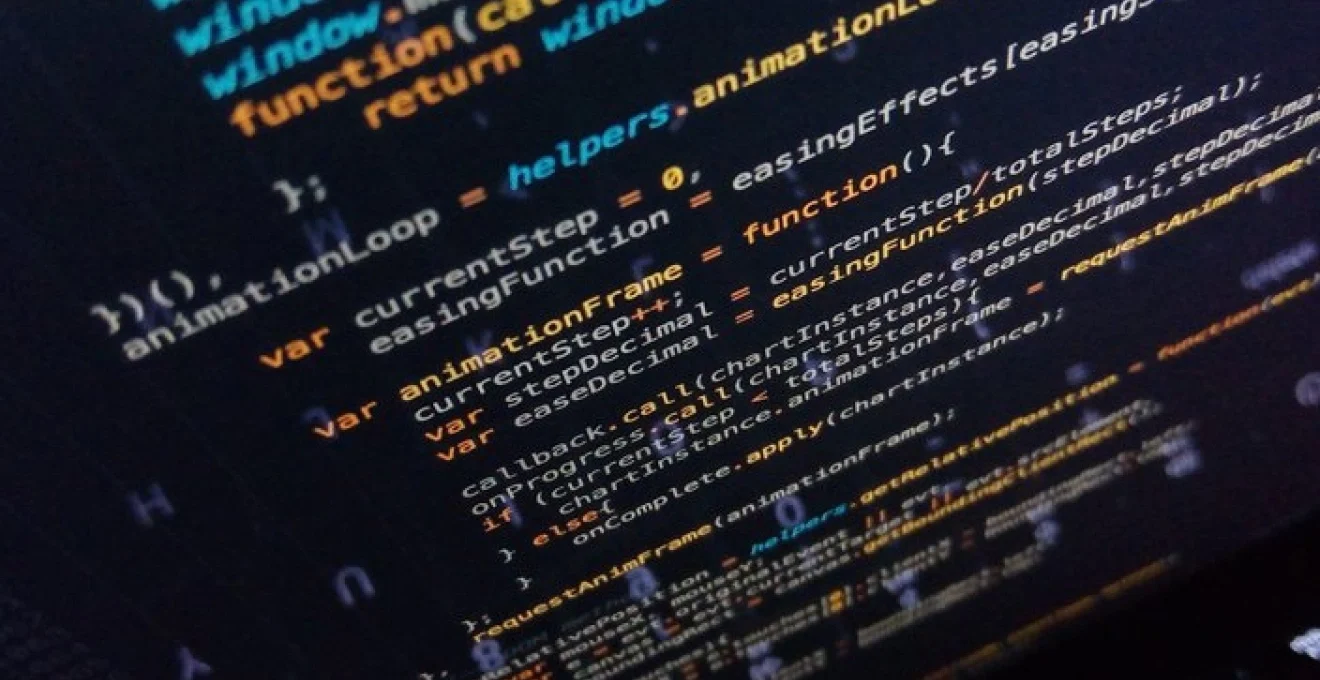
Web development has evolved from simple static pages to complex, interactive applications that power our digital world. The field continues to expand with new frameworks, libraries, and methodologies emerging regularly. For those looking to build a career in tech, web development offers countless opportunities with its blend of creative and technical challenges. The demand for skilled developers remains consistently high across industries, with employers seeking professionals who can navigate both frontend and backend technologies with confidence.
Today's web developers need a diverse skill set that encompasses everything from responsive design principles to server architecture and deployment strategies. Modern development practices have shifted toward full-stack proficiency, where developers understand the entire application lifecycle. This comprehensive approach enables teams to build more cohesive products and gives individual developers greater career flexibility and market value.
Full-stack development fundamentals: HTML5, CSS3, and JavaScript ES6+
The foundation of web development rests on three core technologies: HTML5 for structure, CSS3 for presentation, and JavaScript for behavior. These technologies form the bedrock upon which all web applications are built. A thorough understanding of these fundamentals is essential before progressing to more advanced frameworks and libraries. Developers who master these core technologies can troubleshoot issues more effectively and adapt more quickly to new tools as they emerge.
Modern web development requires proficiency in the latest versions of these technologies. HTML5 introduced semantic elements that improve accessibility and SEO. CSS3 brought powerful layout systems like Grid and Flexbox that revolutionized responsive design. JavaScript ES6+ transformed the language with features like arrow functions, promises, and modules that make code more maintainable and efficient.
Mastering semantic HTML5 elements and accessibility standards
Semantic HTML5 goes beyond basic structure by providing meaning to web content. Elements like
, , and
communicate the purpose of content to browsers, search engines, and assistive technologies. This semantic clarity improves accessibility for users with disabilities and enhances SEO performance. Understanding the appropriate use of these elements is fundamental to creating well-structured, accessible websites.
Web accessibility has become increasingly important, with many countries implementing legal requirements for digital accessibility. The Web Content Accessibility Guidelines (WCAG) provide a framework for making web content more accessible to people with disabilities. Implementing these standards involves proper HTML structure, adequate color contrast, keyboard navigation, and screen reader compatibility. Developers must integrate these considerations from the beginning of the development process rather than treating accessibility as an afterthought.
Accessibility is not a feature or a 'nice-to-have' - it's a fundamental aspect of web development that ensures everyone can access digital content regardless of their abilities or the devices they use.
Advanced CSS3 techniques: grid layout, flexbox, and custom properties
CSS Grid and Flexbox have transformed web layout design, providing powerful tools for creating complex, responsive layouts with clean, maintainable code. Grid excels at two-dimensional layouts (rows and columns), while Flexbox specializes in one-dimensional arrangements. Understanding when and how to use each system allows developers to create sophisticated interfaces that adapt seamlessly to different screen sizes and device types.
Custom properties (CSS variables) have introduced a new level of dynamism and maintainability to stylesheets. They enable developers to define reusable values that can be updated throughout a stylesheet from a single location. This centralization makes themes, dark mode implementations, and responsive adjustments much more manageable. Combined with CSS preprocessors like Sass or Less, these techniques create more efficient, modular styling systems.
Advanced CSS also encompasses animations and transitions that enhance user experience when implemented thoughtfully. Performance-optimized animations focus on properties that trigger only composition (opacity and transform) rather than layout recalculations. Understanding these nuances helps developers create smooth, engaging interfaces without compromising performance.
Javascript ES6+ features: promises, Async/Await, and destructuring
Modern JavaScript has evolved significantly with ES6+ introducing features that make the language more powerful and developer-friendly. Promises revolutionized asynchronous programming by providing a cleaner alternative to callback functions. The async/await syntax built upon promises to make asynchronous code even more readable, allowing developers to write asynchronous code that looks and behaves like synchronous code.
Destructuring assignment provides a concise way to extract values from arrays or properties from objects. This feature simplifies code and makes it more readable, especially when working with complex data structures. Other valuable ES6+ features include arrow functions, template literals, spread and rest operators, and default parameter values. Together, these features have transformed JavaScript into a more elegant and powerful language.
Understanding JavaScript's event loop and asynchronous nature is crucial for building performant applications. The single-threaded nature of JavaScript means that long-running operations must be handled asynchronously to avoid blocking the main thread. This knowledge forms the foundation for advanced topics like worker threads, memory management, and optimization techniques that ensure smooth user experiences.
DOM manipulation and browser APIs for dynamic web applications
The Document Object Model (DOM) is the programming interface for HTML documents that represents the page as a tree structure. Efficient DOM manipulation is essential for creating interactive web applications that respond to user input. Modern approaches minimize direct DOM manipulation, instead focusing on state management that drives UI updates. This pattern, popularized by frameworks like React, improves performance by reducing unnecessary DOM operations.
Modern browsers provide powerful APIs that extend web capabilities far beyond traditional page rendering. The Fetch API offers a more powerful and flexible feature set for making HTTP requests compared to older XMLHttpRequest. Other important APIs include Web Storage for client-side data persistence, Geolocation for location-aware applications, Web Workers for background processing, and the Web Audio API for sound manipulation.
Progressive Web Apps (PWAs) leverage service workers and various browser APIs to create experiences that rival native applications. Service workers enable offline functionality, push notifications, and background sync capabilities. Understanding these technologies allows developers to build applications that work reliably regardless of network conditions, providing a more robust user experience across devices.
Backend technologies and Server-Side programming
Backend development focuses on server-side logic, database interactions, and application architecture. While frontend technologies create the user interface, backend systems handle data processing, business logic, and security. A comprehensive understanding of both sides enables developers to build cohesive, efficient applications. The backend landscape includes various languages and frameworks, each with specific strengths for different use cases.
Modern backend development has evolved toward microservices architectures and serverless computing. These approaches break applications into smaller, more manageable components that can be developed, deployed, and scaled independently. This shift requires developers to understand distributed systems concepts, service communication patterns, and cloud infrastructure. Platforms like lewagon.com offer training programs that cover these modern architectural patterns alongside traditional backend development.
Node.js and express framework architecture for RESTful APIs
Node.js revolutionized backend development by bringing JavaScript to the server, enabling developers to use the same language throughout the stack. Its event-driven, non-blocking I/O model makes it particularly well-suited for handling concurrent connections efficiently. This architecture makes Node.js an excellent choice for real-time applications, APIs, and microservices where performance under load is critical.
Express has emerged as the de facto standard framework for Node.js applications. It provides a minimal, flexible structure for building web applications and APIs. Express middleware functions offer a powerful mechanism for processing requests, implementing authentication, handling errors, and more. Understanding middleware patterns is essential for organizing Express applications that remain maintainable as they grow in complexity.
RESTful API design principles guide the development of interfaces that are predictable, scalable, and compatible with web standards. These principles include resource-based URLs, appropriate HTTP methods, statelessness, and hypermedia links. Implementing these patterns correctly leads to APIs that are intuitive for developers to use and maintain. Advanced topics include versioning strategies, authentication mechanisms, rate limiting, and documentation standards like OpenAPI.
Python django and flask for rapid application development
Python has gained tremendous popularity in web development due to its readability, extensive library ecosystem, and versatility across domains. Django and Flask represent two different approaches to Python web development, with Django providing a comprehensive, "batteries-included" framework and Flask offering a lightweight, extensible microframework. Both have thriving communities and are used by major companies worldwide.
Django follows the "don't repeat yourself" principle with its integrated ORM, authentication system, admin interface, and form handling. This comprehensive approach accelerates development for complex applications with common requirements. Django's architecture encourages a clean separation of concerns through its Model-View-Template pattern, making codebases more maintainable as they scale.
Flask provides greater flexibility by offering a minimal core that can be extended with various extensions as needed. This approach gives developers precise control over which components to include, making Flask suitable for microservices, APIs, and specialized applications. Understanding both frameworks allows developers to choose the right tool based on project requirements rather than personal preference.
PHP laravel vs. ruby on rails: framework comparison
PHP powers a significant portion of the web, with Laravel emerging as its most popular modern framework. Laravel combines elegant syntax with powerful features like Eloquent ORM, Blade templating, routing, middleware, and authentication. Its ecosystem includes tools like Forge for deployment, Nova for administration, and Vapor for serverless deployment. Laravel's developer experience and comprehensive documentation have revitalized PHP development.
Ruby on Rails pioneered the convention-over-configuration paradigm that influenced many subsequent frameworks. Rails prioritizes developer happiness and productivity through features like seamless database integrations, scaffolding, testing tools, and asset pipeline. Its opinionated approach enforces best practices and reduces decision fatigue. While Rails has seen declining market share, it remains a productive framework with a mature ecosystem.
Feature | Laravel (PHP) | Ruby on Rails |
---|---|---|
Learning Curve | Moderate | Steeper initial curve |
Performance | Good with PHP 8+ | Requires optimization for scale |
Community Size | Very large | Large but stabilizing |
Job Market | Abundant opportunities | Fewer but often higher-paying |
Choosing between these frameworks involves considering team expertise, project requirements, performance needs, and long-term maintenance. Many of the concepts translate between frameworks, so developers familiar with one can usually adapt to another. Understanding the philosophies and patterns of multiple frameworks broadens a developer's perspective and improves architectural decision-making.
Database integration: MongoDB, PostgreSQL, and MySQL implementation
Database selection significantly impacts application architecture and performance. Relational databases like PostgreSQL and MySQL excel at structured data with complex relationships, while document databases like MongoDB offer flexibility for rapidly evolving schemas. Modern applications often employ multiple database types to address different data requirements, an approach known as polyglot persistence.
PostgreSQL has gained popularity for its robust feature set, including advanced data types, comprehensive indexing options, and strong standards compliance. Its support for JSON data types provides some of the flexibility of document databases while maintaining relational integrity. PostgreSQL's extensibility through custom functions and operators makes it particularly suitable for complex analytical workloads.
Object-Relational Mappers (ORMs) like Sequelize, Prisma, and SQLAlchemy abstract database operations into a more developer-friendly interface. These tools increase productivity by handling common tasks like query building, migrations, and relationship management. However, understanding the underlying SQL remains important for optimizing queries and troubleshooting performance issues that may arise from ORM-generated queries.
Graphql API design patterns and performance optimization
GraphQL has emerged as an alternative to REST APIs, offering clients precise control over the data they receive. This eliminates over-fetching and under-fetching problems common with REST endpoints. GraphQL's strongly typed schema serves as a contract between client and server, improving documentation and enabling powerful developer tools. Major platforms have adopted GraphQL, indicating its maturity as a technology.
Implementing GraphQL effectively requires understanding its resolution model, where each field in a query is resolved independently. This model enables efficient data loading patterns like dataloader for batching database queries. Security considerations include query complexity analysis and depth limiting to prevent malicious queries from overwhelming server resources. These patterns ensure GraphQL APIs remain performant as they scale.
GraphQL subscriptions extend the query and mutation capabilities by enabling real-time updates. This feature leverages WebSockets or other persistent connection technologies to push data to clients when relevant events occur. Understanding when to use subscriptions versus traditional polling approaches is important for designing responsive, efficient applications.
Modern frontend frameworks and libraries
Frontend frameworks have transformed web development by providing structured approaches to building complex user interfaces. These frameworks offer solutions for common challenges like state management, component composition, and performance optimization. Understanding the core principles behind these frameworks—rather than just their syntax—prepares developers to adapt as the landscape evolves. Each framework has a unique philosophy and set of trade-offs that make it suitable for different projects.
The rise of component-based architecture has been one of the most significant shifts in frontend development. This approach breaks interfaces into reusable, self-contained components that manage their own state and rendering. Components improve code organization, reusability, and testability. This paradigm is now universal across major frameworks, though the implementation details differ between React, Vue, Angular, and others.
React component architecture and redux state management
React introduced a declarative approach to UI development where interfaces are expressed as functions of state. This paradigm shift simplified mental models for building complex UIs by focusing on "what" should be rendered rather than "how" to update the DOM. React's virtual DOM reconciliation process optimizes rendering performance by minimizing actual DOM manipulations. Understanding this process helps developers write more efficient components.
Component composition forms the foundation of React applications, with data flowing from parent to child components through props. This unidirectional data flow makes applications more predictable and easier to debug. Higher-order components and render props extend this pattern to enable code reuse for cross-cutting concerns like data fetching, authentication checks, and layout containers. Modern React has further refined these patterns with hooks that allow function components to use state and other React features.
Redux provides a predictable state management solution for larger applications where component state becomes unwieldy. Its core principles—single source of truth, read-only state, and pure reducer functions—create a clear data flow that's easier to reason about. While Redux adds some boilerplate code, tools like Redux Toolkit have significantly streamlined the implementation. Alternative state management approaches include Context API for simpler cases or libraries like Recoil and Jotai for more specialized needs.
Vue.js composition API and vuex for scalable applications
Vue.js has gained popularity for its gentle learning curve and flexible integration options. It can be adopted incrementally, from enhancing specific parts of an existing page to building comprehensive single-page applications. Vue combines reactive data binding with a template syntax that feels familiar to developers with HTML experience. This approachability makes Vue an excellent choice for teams transitioning from traditional web development to modern frontend frameworks.
The Composition API introduced in Vue 3 represents a significant evolution in how Vue components are organized. Unlike the Options API that separates code by type (data, methods, computed), the Composition API groups code by logical concern. This approach improves code reuse through composable functions and scales better for complex components. Understanding both APIs allows developers to choose the most appropriate style for each situation.
Vuex provides centralized state management for Vue applications through a similar architecture to Redux, with state, mutations, actions, and getters. Vue's reactivity system integrates seamlessly with Vuex, automatically updating components when state changes. For larger applications, Vuex modules offer a way to organize related state and logic. The Pinia library represents the next evolution of Vue state management with a simpler API and improved TypeScript support.
Angular TypeScript integration and RxJS observables
Angular provides a comprehensive platform for building enterprise-scale applications with integrated solutions for routing, forms, HTTP communication, and testing. Its opinionated structure enforces consistent patterns across projects, making it easier for developers to switch between Angular codebases. This consistency comes with a steeper learning curve but pays dividends for large teams and complex applications.
TypeScript forms an integral part of the Angular ecosystem, providing static type checking that catches errors during development rather than runtime. Angular's extensive use of decorators and dependency injection leverages TypeScript features to create a powerful component model. Understanding TypeScript's advanced types, generics, and utility types helps developers build more robust Angular applications with fewer bugs.
RxJS
Observables is a powerful library for reactive programming that Angular leverages extensively. Observables represent streams of data that can emit multiple values over time. This paradigm is particularly well-suited for handling asynchronous events like user input, HTTP requests, and WebSocket messages. Understanding Observable operators like map, filter, switchMap, and combineLatest enables developers to create sophisticated data transformation pipelines.
Angular's change detection mechanism efficiently updates the view when data changes. This system is built on top of Zone.js, which patches browser APIs to notify Angular when asynchronous operations complete. Understanding how change detection works—and when to optimize it with OnPush strategy—is crucial for building performant applications that remain responsive even with large data sets and complex UI.
Next.js and gatsby for Server-Side rendering and static site generation
Next.js has emerged as the leading React framework for production applications by solving common challenges around routing, data fetching, and rendering strategies. Its hybrid approach supports Server-Side Rendering (SSR), Static Site Generation (SSG), Incremental Static Regeneration (ISR), and client-side rendering within the same application. This flexibility allows developers to choose the optimal rendering strategy for each page based on its specific requirements.
Server-Side Rendering improves performance metrics like First Contentful Paint and SEO by generating HTML on the server for each request. This approach delivers fully rendered content to the browser, eliminating the blank page problem common with client-rendered applications. Next.js simplifies SSR implementation through its data fetching methods like getServerSideProps, which executes on the server before rendering.
Gatsby specializes in static site generation, building HTML, CSS, and JavaScript files at build time rather than runtime. This approach delivers exceptional performance by serving pre-rendered content from CDNs with minimal server involvement. Gatsby's extensive plugin ecosystem and GraphQL data layer facilitate content sourcing from various backends like CMSs, APIs, and markdown files. Recent versions have introduced incremental builds that significantly reduce build times for large sites.
Devops and deployment strategies for web applications
DevOps practices have transformed how web applications are built, tested, and deployed. Continuous Integration and Continuous Deployment (CI/CD) pipelines automate the testing and deployment process, ensuring code quality and reducing the time between writing code and delivering it to users. These automated workflows catch issues early and provide confidence when releasing new features or updates.
Container technologies like Docker have standardized application packaging, making deployments more consistent across different environments. Containers encapsulate application code along with its dependencies, eliminating the "it works on my machine" problem. Orchestration platforms like Kubernetes manage container deployment, scaling, and networking across clusters of machines, enabling highly available, resilient applications.
Infrastructure as Code (IaC) tools such as Terraform and AWS CloudFormation define infrastructure in declarative configuration files. This approach brings software development practices to infrastructure management, including version control, testing, and automation. IaC ensures consistent environments, simplifies the reproduction of configurations, and facilitates disaster recovery planning.
The most effective development teams treat infrastructure with the same care and methodology as application code—testing it, reviewing it, and continuously improving it.
Deployment strategies like blue-green deployments, canary releases, and feature flags minimize risk when releasing new versions. Blue-green deployments maintain two identical environments, switching traffic only after verifying the new version works correctly. Canary releases gradually route traffic to new versions, monitoring for issues before full deployment. Feature flags decouple deployment from release, allowing teams to enable features selectively for specific users or gradually roll them out.
Performance optimization and web vitals metrics
Web performance has direct business impact, with studies consistently showing that faster sites have higher conversion rates, lower bounce rates, and improved user engagement. Google's Core Web Vitals established standardized metrics for measuring user experience: Largest Contentful Paint (LCP) for loading performance, First Input Delay (FID) for interactivity, and Cumulative Layout Shift (CLS) for visual stability. These metrics now factor into search ranking algorithms, making performance optimization both a user experience and SEO concern.
Modern performance optimization requires a comprehensive approach spanning both frontend and backend systems. Frontend optimizations include code splitting to load only necessary JavaScript, tree shaking to eliminate unused code, image optimization techniques like WebP format and responsive images, and implementing effective caching strategies. Server-side optimizations focus on response time, database query efficiency, and leveraging CDNs to distribute content closer to users.
Performance budgets establish quantifiable limits on metrics like page weight, load time, and script execution. These budgets create accountability and prevent performance regressions as features are added. Automated performance testing integrated into CI/CD pipelines can alert teams when changes negatively impact key metrics, allowing issues to be addressed before they reach production.
Mobile performance deserves special attention, as mobile devices often have less processing power and more variable network conditions. Techniques like adaptive serving deliver appropriately sized resources based on device capabilities and connection quality. Progressive enhancement ensures core functionality works even in challenging conditions, with enhanced features available when supported.
Career pathways and specialized roles in web development
The web development field offers diverse career paths beyond the traditional frontend/backend dichotomy. Specialized roles have emerged that focus on specific aspects of the development process. Frontend engineers may specialize further as UI developers focused on component systems, accessibility specialists ensuring inclusive experiences, or animation experts creating engaging interactions. Backend specialists might focus on API design, database optimization, or cloud architecture.
Full-stack developers remain valuable for their comprehensive understanding of both client and server technologies. This versatility is particularly important in startups and smaller teams where resources are limited. As applications grow in complexity, however, specialization often becomes necessary. The most effective developers balance depth in their primary area with sufficient breadth to collaborate effectively across specialties.
DevOps engineering has emerged as a distinct career path focusing on the intersection of development and operations. These professionals build and maintain the infrastructure, automation, and monitoring systems that support modern web applications. Site reliability engineers (SREs) apply software engineering principles to operations, creating scalable and reliable systems through automation rather than manual intervention.
Career advancement in web development typically follows two tracks: technical or managerial. The technical track leads to senior developer, architect, and principal engineer roles with increasing scope and complexity of technical decisions. The management track progresses through team lead and engineering manager positions, focusing more on process, people, and project management. Some organizations offer dual-track promotion paths that recognize the value of both technical and leadership contributions.
Continuous learning is essential in web development regardless of specialization or experience level. The field evolves rapidly, with new frameworks, tools, and best practices emerging regularly. Successful developers cultivate learning habits through a mix of formal education, personal projects, open-source contributions, and community involvement. This ongoing investment in skills development ensures long-term career viability and opens doors to new opportunities.
Web development certifications provide structured learning paths and credential validation, though their importance varies by employer. More valuable than any single certification is a strong portfolio demonstrating practical application of skills. Contributing to open-source projects offers both learning opportunities and visibility within the developer community. Building and maintaining side projects showcases initiative and provides a sandbox for experimenting with new technologies.
Remote work has become increasingly common in web development, offering geographic flexibility and access to a global job market. This shift requires additional skills in self-management, digital communication, and remote collaboration tools. Developers who excel in distributed teams combine technical expertise with strong written communication, time management, and the ability to work autonomously while remaining connected to team objectives.
The most successful web developers combine technical skills with business understanding, seeing beyond code to the product and user needs. This perspective enables them to make better technical decisions aligned with business goals and communicate effectively with non-technical stakeholders. As web applications become increasingly central to business operations, this holistic approach to development becomes ever more valuable.